[react-testing-library] 쿼리의 우선순위
글 작성자: 망고좋아
반응형
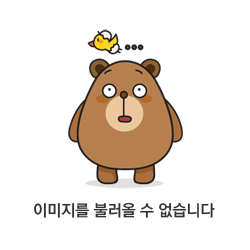
🎯 쿼리의 우선순위
- 유저가 페이지를 이동하는 방식에 가까운 쿼리일수록 우선순위가 높다.
- 접근성 높은 HTML을 작성할수록 테스트가 용이한 코드가 된다.
📝 ByRole (제일 높음)
- accessibility tree에 있는 요소들을 기준으로 원소를 찾는다.
- 유저가 웹 페이지를 사용하는 방식을 가장 닮은 쿼리
- 동일한 role을 가진 경우, accessible name을 이용해 원소를 구별한다.
- accessible name이란 원소의 특징을 나타내는 이름이다.
- 임의로 role 혹은 aria-*을 부여하는 것을 지양한다.
- 자주 사용되는 Role : button, checkbox, listitem, heading, img, form, textbox, link
- 자주 사용되는 accessible name
- button - 텍스트
- label - 텍스트
- a - 텍스트
- img - alt 텍스트
function TestForm() { const formRef = useRef(); const handleSubmit = (e) => { e.preventDefault(); formRef.current.reset(); }; return ( <form onSubmit={handleSubmit} ref={formRef}> <label htmlFor="username">Username</label> <input id="username" type="text" name="username" /> <input type="submit" value="Submit" /> </form> ) } // ---- import userEvent from "@testing-library/user-event"; import { render, screen } from "@testing-library/react"; test('제출 버튼을 찾아 클릭하면, Username 인풋이 비워진다.', () => { const { getByRole } = render(<TestForm />) const usernameInput = getByRole('textbox', { name : 'Username' }) const submitButton = getByRole('button', { name : 'Submit' }) userEvent.type(usernameInput, "test username") userEvent.click(submitButton) expect(usernameInput).toHaveValue("") // 제출버튼 클릭하고 form이 reset 되었는지 테스트 })
📝 Text
- 유저가 볼 수 있는 Text 값을 기준으로 쿼리를 찾는다.
ByLabelText
: label과 연관된 원소를 찾는다ByPlaceholderText
: placeholder와 연관된 원소를 찾는다.ByText
: 주어진 Text와 연관된 원소를 찾는다.ByDisplayValue
: input, textarea, select 등의 value를 기준으로 원소를 찾는다.
import userEvent from "@testing-library/user-event"; import { render } from "@testing-library/react"; test('제출 버튼을 찾아 클릭하면, Username 인풋이 비워진다.', () => { const { getByLabelText, getByText } = render(<SimpleTestForm />); const usernameInput = getByLabelText("Username"); const submitButton = getByText("Submit"); userEvent.type(usernameInput, "test username"); userEvent.click(submitButton); expect(usernameInput).toHaveValue(""); })
📝 semantic queries
- 유저에게 보이지 않지만 접근성 스펙에 적합한 alt, title을 이용하여 원소를 검색한다.
ByAltText
: img, area, input 등의 alt 속성으로 원소를 검색ByTitle
: title 속성으로 원소를 검색
📝 Test ID
- data-testid 속성을 원하는 원소에 지정하고, 쿼리를 이용해 찾는다.
- 유저가 해당 속성을 기반으로 화면의 요소를 찾는 게 아니므로 우선순위가 낮다.
- 다른 쿼리로 테스트를 작성할 수 없을 때 이 쿼리를 백도어로 활용한다.
import userEvent from "@testing-library/user-event"; import { render } from "@testing-library/react"; test('제출 버튼을 찾아 클릭하면, Username 인풋을 비운다.', () => { const { getByTestId } = render(<SimpleTestForm />); const usernameInput = getByTestId("username-input"); const submitButton = getByTestId("submit-button"); userEvent.type(usernameInput, "test username"); userEvent.click(submitButton); expect(usernameInput).toHaveValue(""); })
반응형
'프로그래밍 > testing' 카테고리의 다른 글
[react-testing-library] 테스트 코드 작성 시 Tip (0) | 2022.03.02 |
---|---|
[react-testing-library] 유저 이벤트 (0) | 2022.01.17 |
[react-testing-library] react-testing-library란? (0) | 2022.01.17 |
[Jest] Async assertion (0) | 2022.01.17 |
[Jest] Assertion Matchers 활용 (0) | 2022.01.17 |
댓글
이 글 공유하기
다른 글
-
[react-testing-library] 테스트 코드 작성 시 Tip
[react-testing-library] 테스트 코드 작성 시 Tip
2022.03.02 -
[react-testing-library] 유저 이벤트
[react-testing-library] 유저 이벤트
2022.01.17 -
[react-testing-library] react-testing-library란?
[react-testing-library] react-testing-library란?
2022.01.17 -
[Jest] Async assertion
[Jest] Async assertion
2022.01.17
댓글을 사용할 수 없습니다.